As a versatile and powerful programming language, JavaScript offers a myriad of functions and features that help create complex, interactive web applications. One key, yet often overlooked, aspect of this toolkit is indexing. Indexing in JavaScript involves accessing specific elements within data structures like arrays and strings, based on their position or “index.”
Understanding JavaScript indexing is crucial for efficiently navigating, manipulating, and extracting information from data structures. In this article, we’ll cover everything you need to know about JavaScript indexing, starting from the basics and advancing toward more sophisticated techniques and practical applications.
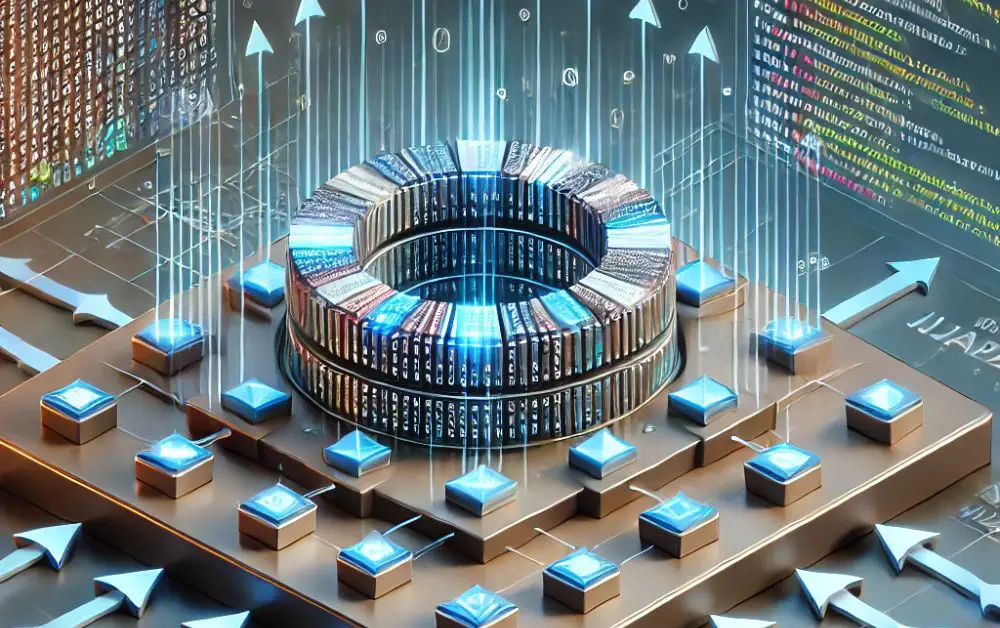
What is Indexing in JavaScript?
In programming, indexing refers to the ability to access individual elements in a collection or data structure based on their location. In JavaScript, two of the most commonly indexed data structures are arrays and strings.
- Arrays: Ordered collections of elements, where each element is assigned a numerical index, starting from zero.
- Strings: Ordered sequences of characters, where each character is similarly indexed, starting at zero.
Basic Indexing in Arrays and Strings
Indexing allows developers to access, manipulate, and retrieve elements from arrays and strings by specifying their position.
Accessing Array Elements
Arrays in JavaScript are zero-indexed, meaning the first element is at position 0
, the second at position 1
, and so on. To access an array element, you simply reference its index:
let fruits = ['Apple', 'Banana', 'Cherry'];
console.log(fruits[0]); // Output: Apple
console.log(fruits[2]); // Output: Cherry
Accessing String Characters
Like arrays, strings in JavaScript can be indexed using numerical positions. Each character in a string is assigned an index starting from 0
.
let greeting = 'Hello';
console.log(greeting[0]); // Output: H
console.log(greeting[4]); // Output: o
Negative Indexing in JavaScript
Unlike some other programming languages, JavaScript does not natively support negative indexing (i.e., accessing elements from the end of the array). However, you can still achieve this using a simple workaround by subtracting the desired index from the array’s length:
let fruits = ['Apple', 'Banana', 'Cherry'];
console.log(fruits[fruits.length - 1]); // Output: Cherry
Modifying Array Elements Using Indexing
JavaScript also allows you to modify the value of an array element using its index:
let fruits = ['Apple', 'Banana', 'Cherry'];
fruits[1] = 'Blueberry';
console.log(fruits); // Output: ['Apple', 'Blueberry', 'Cherry']
This ability to update specific elements is critical when working with dynamic datasets or managing state in web applications.
Advanced Indexing Techniques
Beyond the basics of accessing and modifying individual elements, JavaScript offers more advanced methods for working with indexed data structures.
Iterating Over Indexed Elements
One of the most common operations performed on arrays and strings is iterating over their elements. JavaScript provides several ways to loop through indexed data structures, such as for
, forEach
, and map
.
Using for
Loop
The classic for
loop is a straightforward way to access each element in an array or string by its index.
let fruits = ['Apple', 'Banana', 'Cherry'];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
// Output: Apple, Banana, Cherry
Using forEach
The forEach
method provides a more modern and concise way to iterate over array elements. It accepts a callback function that is executed once for each element.
let fruits = ['Apple', 'Banana', 'Cherry'];
fruits.forEach((fruit, index) => {
console.log(`${index}: ${fruit}`);
});
// Output: 0: Apple, 1: Banana, 2: Cherry
Multi-dimensional Arrays and Indexing
JavaScript supports multi-dimensional arrays, where each element in the array is itself an array. These arrays can be accessed using multiple indices.
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log(matrix[0][2]); // Output: 3
console.log(matrix[2][1]); // Output: 8
Multi-dimensional arrays are useful for representing complex data structures like grids, tables, and matrices.
Destructuring Arrays for Indexed Elements
With array destructuring, you can easily assign values from an array to variables based on their index.
let fruits = ['Apple', 'Banana', 'Cherry'];
let [firstFruit, secondFruit] = fruits;
console.log(firstFruit); // Output: Apple
console.log(secondFruit); // Output: Banana
You can also skip elements during destructuring by leaving placeholders:
let [first, , third] = fruits;
console.log(first); // Output: Apple
console.log(third); // Output: Cherry
Using Array.prototype.findIndex()
The findIndex()
method returns the index of the first element in an array that satisfies a given condition, making it a useful tool for searching indexed data.
let fruits = ['Apple', 'Banana', 'Cherry'];
let index = fruits.findIndex(fruit => fruit === 'Banana');
console.log(index); // Output: 1
Practical Applications of Indexing
Indexing plays a vital role in solving real-world problems in JavaScript development, such as:
- Searching for Data: Indexing allows efficient searches through arrays for specific elements or values, especially in large datasets.
- Manipulating DOM Elements: In web development, indexed access to DOM elements (e.g., form inputs, images) is often used for modifying the content dynamically.
- Data Structures: Many data structures like linked lists, stacks, and queues rely heavily on indexed operations for insertion, deletion, and traversal.
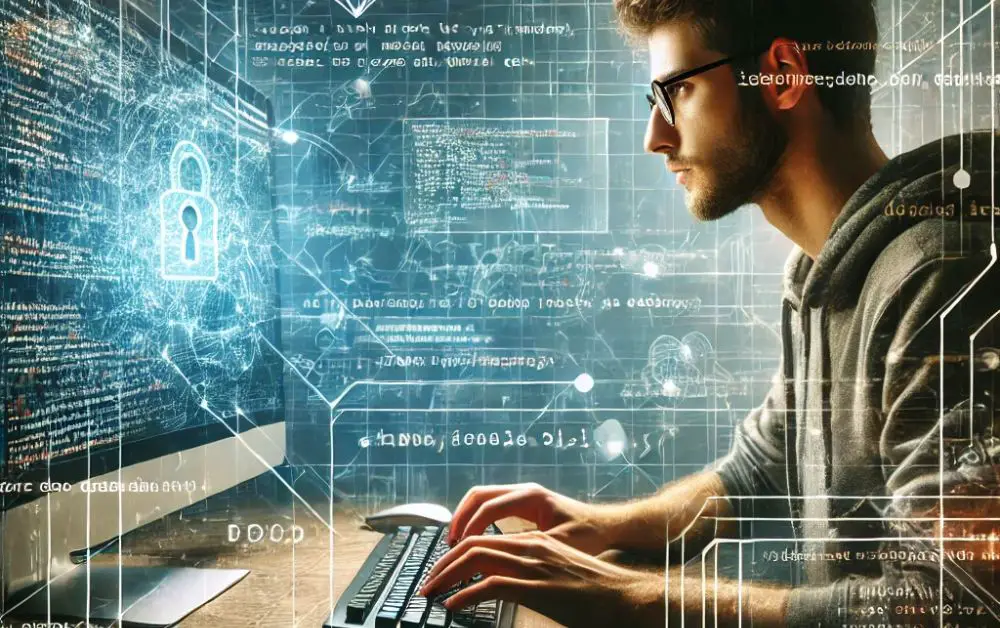
Conclusion
Indexing is a fundamental concept in JavaScript that forms the backbone of data manipulation in arrays and strings. By mastering basic and advanced indexing techniques, developers can navigate, access, and manipulate data with greater efficiency and flexibility.
From simple array indexing to advanced applications like multi-dimensional arrays and findIndex()
, a solid grasp of these concepts enables you to write cleaner, more optimized code, especially when dealing with dynamic or large datasets.
JavaScript’s indexing capabilities are a crucial tool for any developer looking to build interactive and data-driven web applications, so mastering them will undoubtedly enhance your coding skills and make your applications more efficient.