JavaScript indexing is a fundamental concept that every developer encounters when working with data structures. Understanding how indexing works is crucial to manipulating arrays, accessing object properties, or optimizing for search engines. This article explores JavaScript indexing across different contexts, common pitfalls, and best practices.
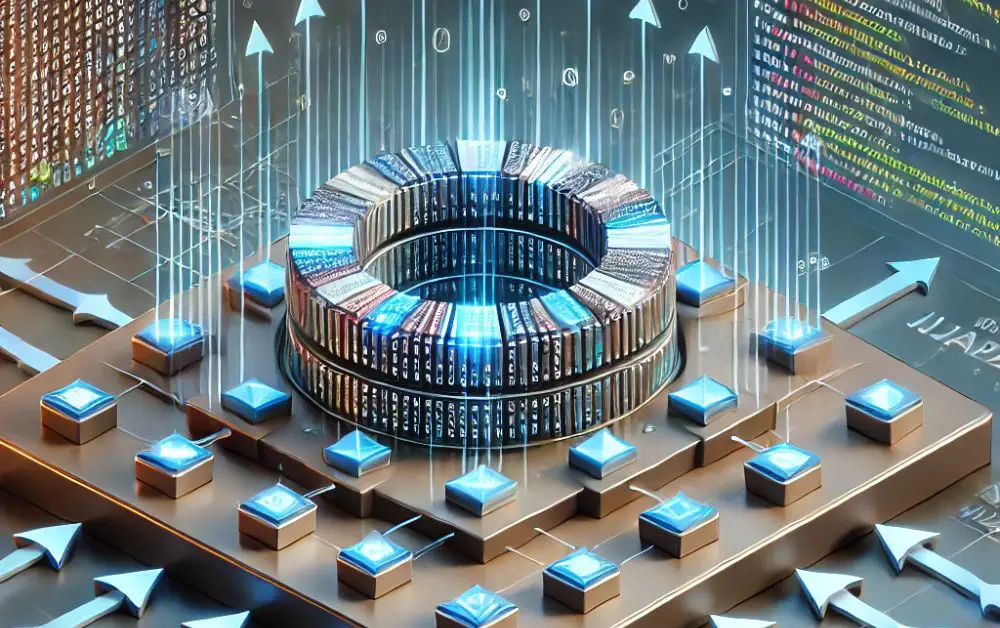
1. Array Indexing: Zero-Based and Dynamic
Arrays in JavaScript are ordered collections that use zero-based indexing, meaning the first element is at position 0
. This contrasts with languages like R or Julia, which use one-based indexing.
let fruits = ['apple', 'banana', 'orange'];
console.log(fruits[0]); // 'apple'
console.log(fruits[2]); // 'orange'
Key Characteristics:
- Dynamic Sizing: Arrays resize automatically when elements are added/removed.
- Sparse Arrays: JavaScript allows gaps in arrays (e.g.,
let arr = []; arr[3] = 'value';
), wherearr.length
becomes4
, but indexes 0–2 areundefined
. - Methods Affecting Indexes: Methods like
push()
,pop()
,shift()
, andsplice()
directly impact array indexes. For example,shift()
removes the first element, shifting all other elements down by one index.
Negative Indexing with at()
ES2022 introduced at()
, supporting negative indexes to access elements from the end:
console.log(fruits.at(-1)); // 'orange' (same as fruits[fruits.length - 1])
2. Object Properties: Key-Value Pairs
Objects use keys (strings or symbols) to index values, unlike arrays. While traditional objects don’t guarantee order, modern ES6+ implementations preserve insertion order:
let user = { name: 'Alice', age: 30 };
console.log(user['name']); // 'Alice'
Maps for Ordered Key-Value Storage
ES6’s Map
offers better performance for frequent additions/removals and maintains insertion order:
let map = new Map();
map.set('name', 'Bob');
console.log(map.get('name')); // 'Bob'
3. String Indexing: Immutable Characters
Strings behave like read-only arrays, where each character is accessible via index:
let greeting = 'Hello';
console.log(greeting[1]); // 'e'
console.log(greeting.charAt(4)); // 'o'
Attempting to modify a character directly (e.g., greeting[0] = 'M';
) fails silently because strings are immutable.
4. Search Engine Indexing Challenges
JavaScript-heavy websites (e.g., SPAs) can face SEO issues because search engine crawlers may struggle to execute client-side JavaScript. Solutions include:
- Server-Side Rendering (SSR): Tools like Next.js pre-render pages on the server.
- Prerendering Services: Platforms like Prerender generate static HTML for crawlers.
- Dynamic Rendering: Serve static HTML to bots and client-side JS to users.
5. Common Pitfalls and Best Practices
- Off-by-One Errors: Remember that arrays start at
0
.
// Looping safely:
for (let i = 0; i < arr.length; i++) { ... }
- Avoid Sparse Arrays: They consume memory and cause unexpected
undefined
values. - Use Arrays for Ordered Data: Opt for arrays (or TypedArrays for numeric data) instead of objects when order matters.
- Check Boundaries: Use optional chaining (
arr?.[index]
) to avoid errors when accessing uncertain indexes.
6. Performance Considerations
- Prefer
for
Loops Overfor...in
: Index-basedfor
loops are faster for array iteration. - TypedArrays: For numeric data (e.g., WebGL), use
Uint8Array
orFloat64Array
for memory efficiency.
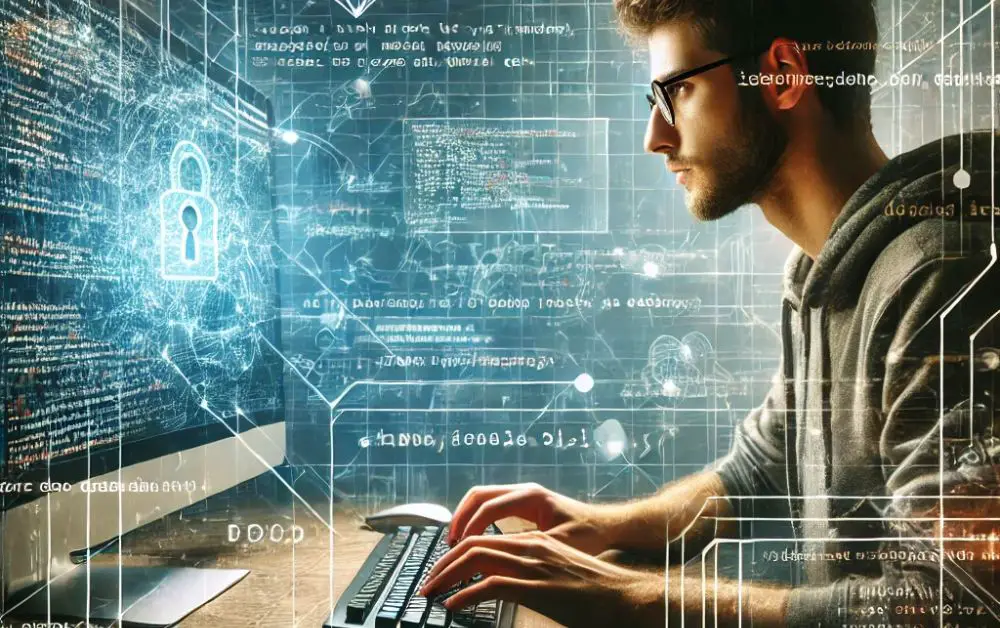
Conclusion
JavaScript indexing underpins how developers interact with arrays, objects, and strings. Key takeaways include:
- Arrays use zero-based, dynamically sized indexes.
- Objects and Maps offer key-based indexing with ordered guarantees in modern JS.
- Strings are immutable but indexable.
- SEO requires special attention for JavaScript-rendered content.
By mastering these concepts, you’ll write cleaner, more efficient code and avoid common indexing pitfalls.
Best Web Development Tools in 2025: Top Picks for Developers